题目链接:https://leetcode-cn.com/problems/longest-common-prefix/
题目描述
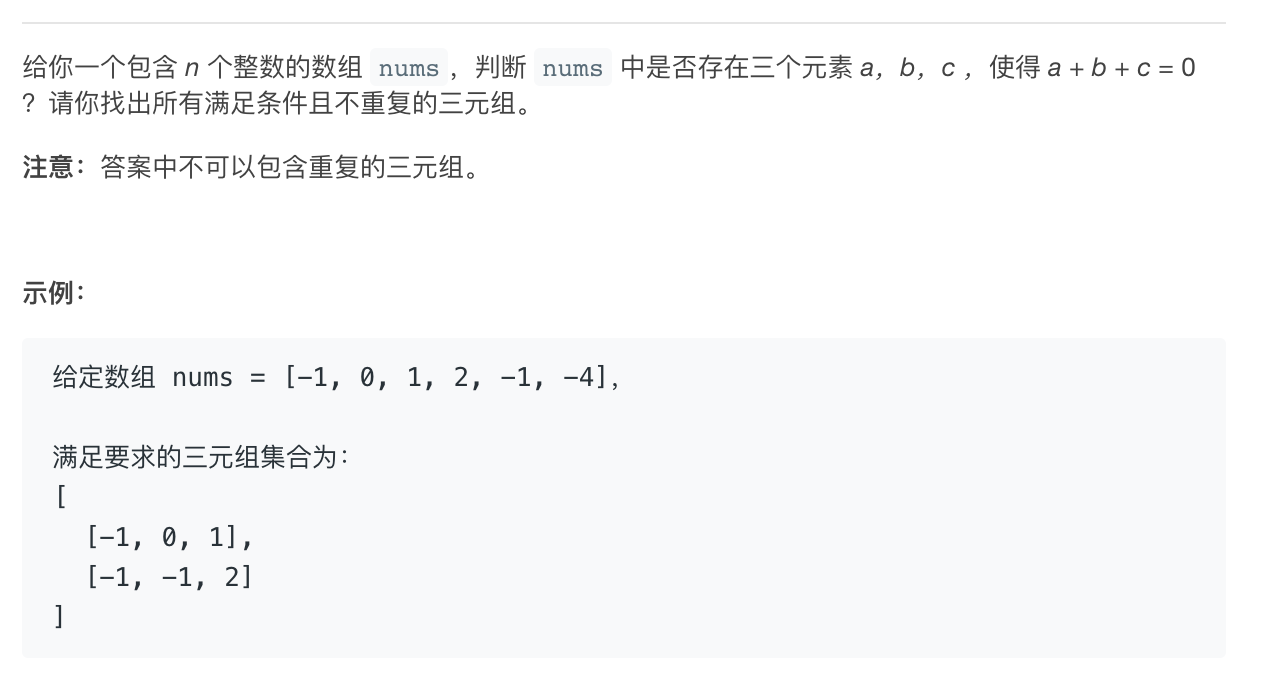
分析
1. 循环
代码实现
public enum Q14 {
instance;
public String longestCommonPrefix(String[] strs) {
if(strs==null||strs.length==0){
return "";
}
// 先获取最短字符串
int min=Integer.MAX_VALUE;
for(String str:strs){
min=min>str.length()?str.length():min;
}
// 对比每个字符相等
int point=0;
for(int i=0;i<min;i++){
boolean f=true;
for(int j=0;j<strs.length-1;j++){
if(strs[j].charAt(i)!=strs[j+1].charAt(i)){
f=false;
}
}
if(!f){
break;
}else {
point++;
}
}
return point==0?"":strs[0].substring(0,point);
}
public static void main(String[] args) {
// assert fl
String[] strs1 = new String[]{"flower","flow","flight"};
System.out.println(Q14.instance.longestCommonPrefix(strs1));
// assert
String[] strs2 = new String[]{"dog","racecar","car"};
System.out.println(Q14.instance.longestCommonPrefix(strs2));
}
}